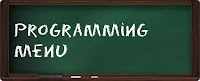
Focusing on the goal of our Java course, and show that it is doing useful things, we show a utility method that simply display messages on the screen.
Let us use exercise 9 on simple outputs.
Basically, we use our expertise in loops (do ... while), the command switch (to choose from the menu options) and 5 methods.
A method for each option (include, change, delete, search) and another method that shows this menu of options.
Because options are numbers, we use an integer type, called 'option' to receive user choices.
As we want the menu to appear at least once, we use the do ... while looping.
By entering this loop, the menu is displayed with the command 'menu();' that calls the method that displays the menu.
Soon after, the program waits for user input. Depending on what was typed, the specific method - include(), change(), delete() or search() - is selected by the switch.
The program ends only if the user enters 0.
Java code of this application:
import java.util.Scanner;
public class menu {
public static void menu(){
System.out.println("\tCustomer base");
System.out.println("0. End");
System.out.println("1. Include");
System.out.println("2. Change");
System.out.println("3. Delete");
System.out.println("4. Search");
System.out.println("Option:");
}
public static void include(){
System.out.println("You are in include() method.");
}
public static void change(){
System.out.println("You are in change() method.");
}
public static void delete(){
System.out.println("You are in delete() method.");
}
public static void search(){
System.out.println("You are in search() method.");
}
public static void main(String[] args) {
int option;
Scanner input = new Scanner(System.in);
do{
menu();
option = input.nextInt();
switch( input ){
case 0:
System.out.println("Exiting...");
break;
case 1:
include();
break;
case 2:
change();
break;
case 3:
delete();
break;
case 4:
search();
break;
default:
System.out.println("Invalid option.");
}
} while(opcao != 0);
}
}
No comments:
Post a Comment